Develop new features with iterative coding and feature flags
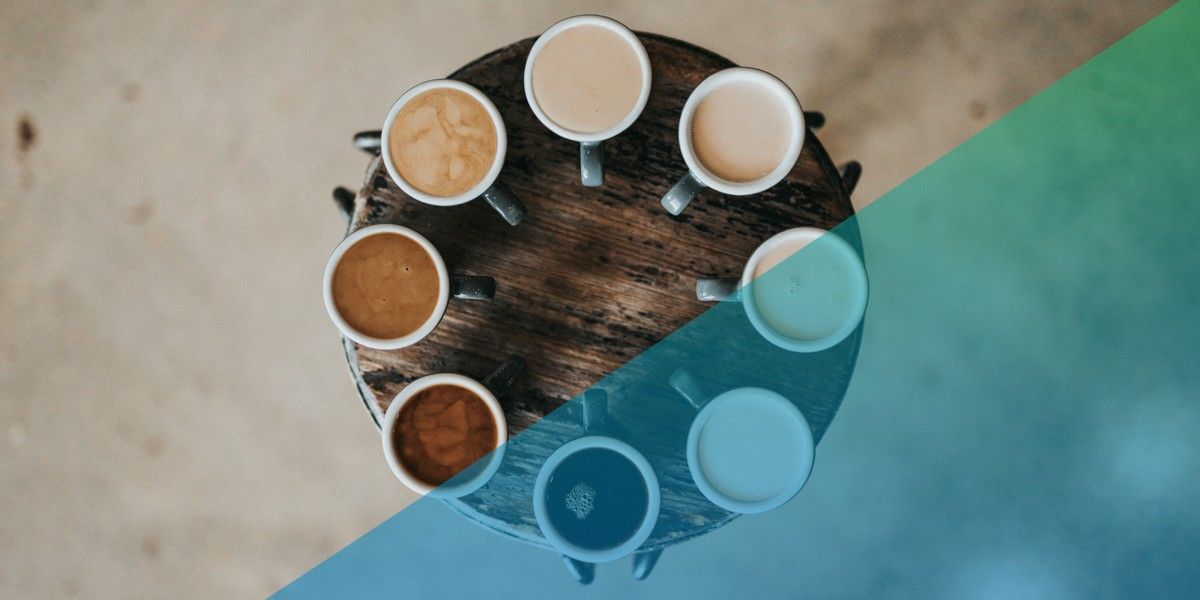
A while ago we wrote about iterative coding, which is a way to develop your project in short cycles, each one producing a working and usable artifact.
This approach can be used in a fractal fashion and applied each time you develop a new feature on an existing and working project.
First thing we do, we identify all supporting changes:
- create new services
- add new methods in existing services
- change entities structure
We look for all modifications that do not change the existing behaviour of the project, so they can be coded, tested and released separately. Depending on how big are each of these supporting tasks, we may decide to make several releases. We will add tests for these supporting changes and make sure we did not introduce any regression bug.
The idea is always to introduce the minimum amount of change at any given release, because if anything goes wrong we have a smaller codebase to scan for defects. Also, this is good for planning: we may not always have time to code a big feature in a single session, and small tasks are easier to fit into our planning.
Following along the same guidelines, another great idea are feature flags. In a few words, this means that the new feature is already in production but dormant, unless a specific configuration switch is enabled.
Example
Let us make an example. Imagine we have a product list that is sorted alphabetically. Now we want to introduce a smarter algorithm that combines user history, best-selling products, and some other factors to show each user a customized list. First thing we would do, is to create the service which retrieves the customized product list. let’s call it CustomProductListBuilder. This is a support task: the service will not be used anywhere yet and will not change the existing behaviour, so we can test it and release it in production. Next, we will create a feature flag so we can make sure the new algorithm is not actually used unless we enable that switch.
public function indexAction() { if ($this->getParameter('custom_product_list')) { return $this->get('CustomProductListBuilder')->getProducts($this->getUser()); } return $this->get('ProductRepository')->findBy([], ['name' => 'asc']); }
This flag allows us to:
* test the feature on a separate stage / test environment
* quickly turn it on / off in production e.g. to see that the customized list actually makes sense or to disable it in case anything is wrong
* with a slightly more advanced feature check, to enable it only for some users
Conclusions
Our target is always to have our code in production as soon as possible, as long as it’s tested. We do this by breaking each feature development in smaller tasks and by using feature flags.
If you want to know more about it, feel free to contact us by using the contact form below!