API Platform: Modernising API Development
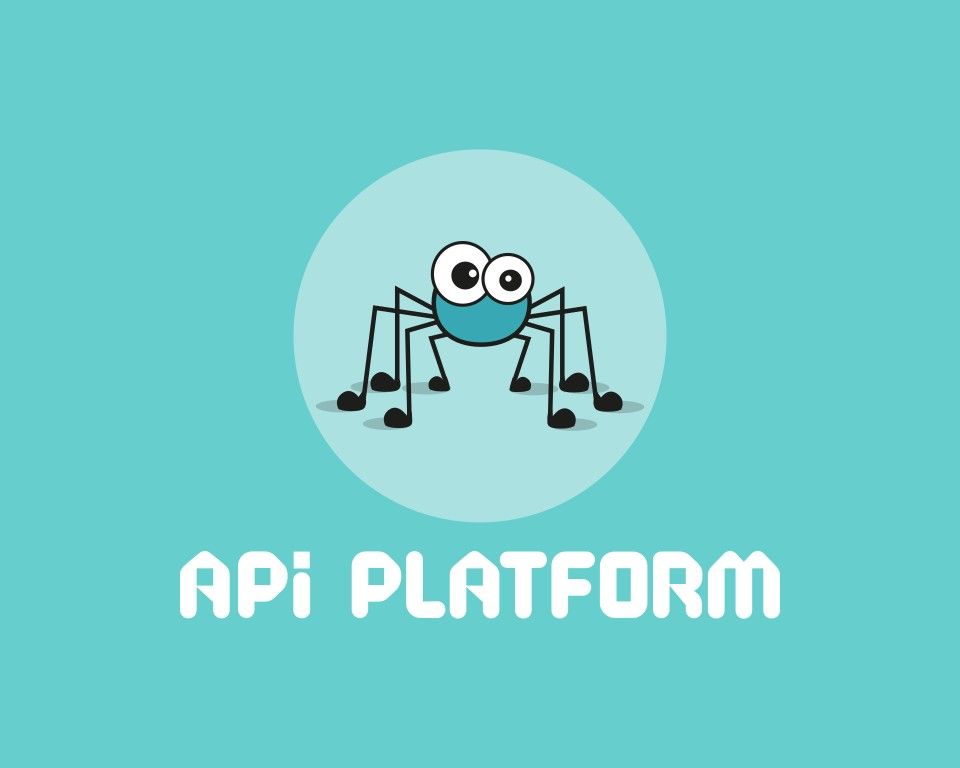
What is API Platform
API Platform is a powerful full-stack framework designed to simplify the creation of web APIs. Built on top of Symfony, it offers an ‘API-first’ approach that enables the development of modern APIs following the latest standards such as JSON-LD, HYDRA and OpenAPI. Its distinguishing feature is the ability to automatically generate CRUD operations, interactive documentation and client interfaces from simple PHP classes.
As Fabien Potencier, creator of Symfony, put it: ‘API Platform is the most advanced API platform, in any framework or language’. This statement is supported by the wealth of functionality that the framework offers out-of-the-box:
- Automatic generation of CRUD operations
- Support for REST and GraphQL
- Interactive documentation with OpenAPI (Swagger)
- Integrated validation system
- Advanced management of relations between entities
- Support for real-time via the Mercure protocol
- Integrated HTTP caching system
- Automatic client generation (React, Vue.js, …)
The API Platform Philosophy
The API Platform Philosophy emerged from observing the web’s evolution over a quarter century, shaping its approach to modern development challenges. It recognizes that Progressive Web Apps built with technologies like React and Vue.js have become the new standard for web applications, while acknowledging the shift toward mobile-first development as users increasingly access the internet through mobile devices.
The platform embraces the Semantic Web movement, supporting Linked Data and Schema.org initiatives to enable machine-readable structured data. It also leverages the performance capabilities of modern protocols like HTTP/2 and HTTP/3 to optimize web application delivery.
The Mercure Protocol: Real-time under the bonnet
One of the most innovative features of API Platform is its native integration with the Mercure protocol, which enables real-time functionality in APIs. Mercure allows real-time updates to be sent to connected clients (web browsers, mobile applications) using Server-Sent Events (SSE).
Here is an example of how to implement Mercure support in an entity:
<?php
namespace App\Entity;
use ApiPlatform\Metadata\ApiResource;
use Doctrine\ORM\Mapping as ORM;
#[ApiResource(
mercure: [
'private' => true,
'topics' => [
'@=iri(object)',
'@=iri(object.getOwner()) ~ "/?topic=" ~ escape(iri(object))'
]
]
)]
#[ORM\Entity]
class Notification
{
#[ORM\Id, ORM\Column, ORM\GeneratedValue]
private ?int $id = null;
#[ORM\Column(type: 'text')]
private string $message = '';
#[ORM\ManyToOne]
private ?User $owner = null;
// ... getters and setters
}
Advantages in Adoption
API Platform has demonstrated significant value in enterprise environments through several key capabilities. The platform automates code and interface generation, which reduces development cycles and deployment timelines. Its standardized architecture minimizes boilerplate code and establishes consistent development patterns, leading to more sustainable codebases.
Development teams benefit from comprehensive auto-generated documentation and widely adopted standards, which streamlines both onboarding and ongoing development. The platform’s structured approach enables teams to maintain consistency as they scale, while its performance optimizations and reduced maintenance requirements help control operational costs. Additionally, its framework-agnostic design provides the technical flexibility to adapt to various project requirements and front-end technologies.
Practical Example in Symfony
Here is a complete example of an API Platform entity with validation and relations:
<?php
namespace App\Entity;
use ApiPlatform\Metadata\ApiResource;
use ApiPlatform\Metadata\Get;
use ApiPlatform\Metadata\GetCollection;
use ApiPlatform\Metadata\Post;
use Doctrine\ORM\Mapping as ORM;
use Symfony\Component\Validator\Constraints as Assert;
#[ApiResource(
operations: [
new Get(),
new GetCollection(),
new Post()
],
paginationItemsPerPage: 20
)]
#[ORM\Entity]
class Product
{
#[ORM\Id]
#[ORM\GeneratedValue]
#[ORM\Column]
private ?int $id = null;
#[ORM\Column]
#[Assert\NotBlank]
#[Assert\Length(min: 3, max: 255)]
private string $name = '';
#[ORM\Column(type: 'text')]
#[Assert\NotBlank]
private string $description = '';
#[ORM\Column]
#[Assert\PositiveOrZero]
private float $price = 0.0;
#[ORM\ManyToOne(targetEntity: Category::class, inversedBy: 'products')]
#[Assert\NotNull]
private ?Category $category = null;
// ... getters and setters
}
Future Perspectives
In our company context, we currently run a Yii2-based project with numerous CRUD sections. As discussed in a previous article: A nice little fine PHP framework called Yii, upgrading to Yii3 is not currently a path we intend to pursue with any certainty. Instead, an interesting prospect could be a gradual and controlled refresh using Symfony with API Platform.
This approach would allow us to modernise our code base through a side-by-side migration process, where new features could be developed using the power of API Platform.
Are you considering API Platform for your project? We can support you on your API modernisation journey. Contact us to discuss your project and have a look at our technologies page to find out what other tools we work with on a daily basis.