Refactoring your code
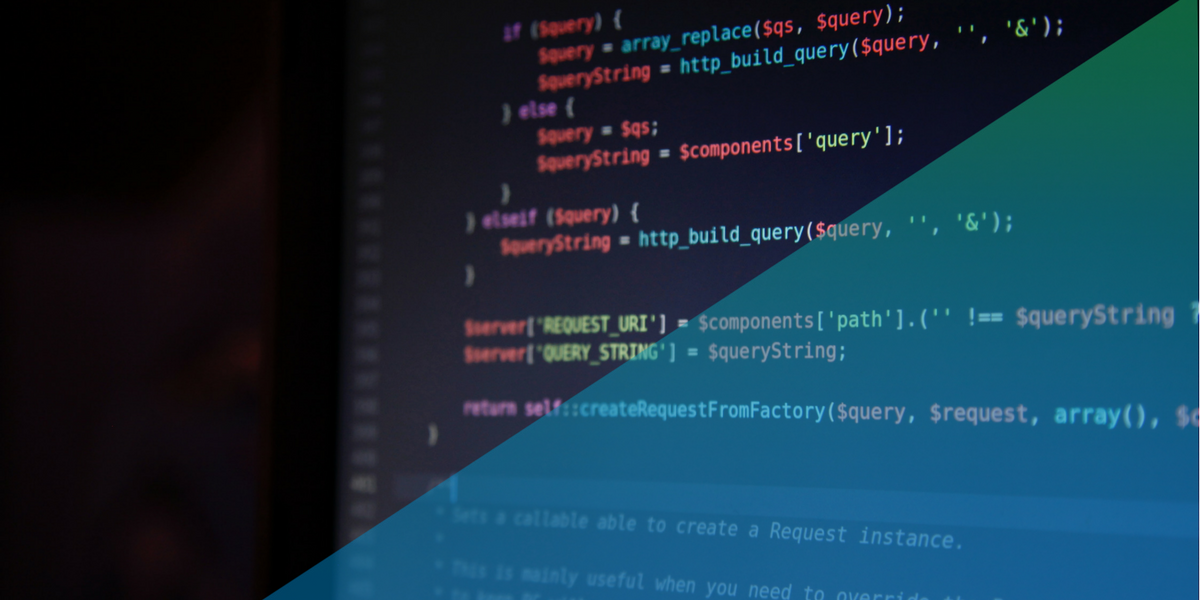
Refactoring is an important skill in any successful developer tools.
You should do it now, there are known procedures to do it and it all really boils down to a few important principles.
Let’s review them.
What’s refactoring?
Refactoring, as Martin Fowler puts it, is the process of “improving the design of existing code“.
It is when you take your code and make it better without (necessarily) adding features or fixing bugs.
So, in this context, “better” means that it becomes easier to understand, extend or debug.
The bad smell vs. the WTF rate
To a different level, all code needs refactoring. I guess perfection does not exist.
But of course there is some code that needs it more than other.
There are two common ways to measure the urgency of refactoring in any code.
One is the bad smell. In the book about it by Martin Fowler and others, the bad smell is defined as a series of code structures or design choices that makes other developers turn away in disgust.
It’s something that you need to have a nose for, and that comes with experience: pretty soon you start to separate the complex code (which is above your current level of comprehension) from the bad code (which unfortunately you understand quite enough to decide that it sucks).
The other measurement is the WTF factor. It’s not easy to describe it with plain and elegant words, but it goes more or less like this: you make other developers read some code. The higher the number of “WTF” you hear, the more that code needs refactoring.
This second is good because you don’t really need a high level of experience; a perfectly written code should be quite clear even to a novice.
When do you refactor?
Every day.
And please let me make that clear: every day.
I can assure you – by personal experience – that it’s not generally a good idea to set apart a fixed period to refactor, and then go back to code new features, and then back again to refactor, and so on.
Instead, everytime you’re fixing a bug or adding a feature, and you notice something is wrong in the code, you just go ahead and fix it.
Don’t let your managers fool you into “we will make refactoring when we have time”: when you need to add a lot of features, that’s exactly the time when you need refactoring the most.
The sacred principles of refactoring
You may write a book about refactoring – and in fact, Martin Fowler did – but let’s review just some really basic principles.
- You test
Refactoring is dangerous. You take existing, working code and start messing around with it. If you can’t test afterwards that everything is fine, you’re putting yourself in a very delicate position.
- You choose sensible names
Most of refactoring is about making your code more easy to understand, and most of that is about choosing adequate names for your variables and methods. Never, ever underestimate this.
- You use short methods
Every long method is a pain to understand and to fix. So what you do is, you break it into smaller methods, with clear names, and that is already a big improvement.
Do not fear to have many methods. When you read code you don’t read it sequentially, you follow the workflow. That will be easier if your methods are short and sensibly named (did I mention that already?).
- You avoid duplicated code
Try hard not to have the same (or very similar) code spread throughout your project. There are many ways to fix this, from helpers to inheritance to composition, and reducing lines of code is always a win.
- You really think about your conditionals
If, else, switch are the parts of your code where most of the complexity resides. It’s not easy to follow the flow in conditionals. So, you really try to reduce conditionals. Or, you make them more clear by calling a method for each leg instead of having 200 lines of code between the “if” and the “else”.
- You learn about available libraries and functions
No need to rewrite a json parsing method since php json_decode does exactly that. No need to use a low level fsckopen call since curl already takes care of much more. You use a query builder whenever it’s appropriate, since it’s more easy to comprehend than a 20 lines query with random spacing.
Best practice
Refactoring should become one of your most applied best practices.
It will help you and others working on the code, and that alone should make you feel better.
There is definitely something Zen about refactoring.
You are one with your code. If your code is not good, you are not good.