Yii query optimization: small changes, big impact
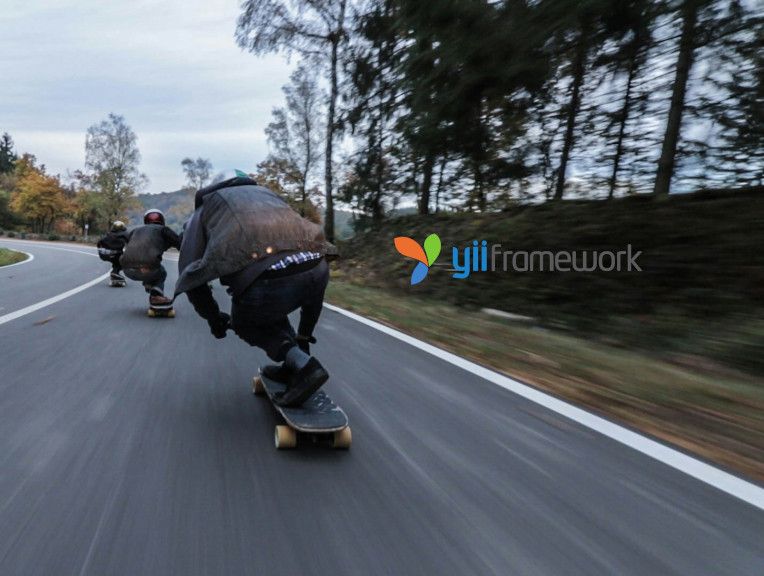
At Refactory, we believe that attention to detail makes the difference between good code and great code. A recent optimization in one of our Yii2 projects perfectly illustrates this philosophy.
We encountered a common pattern in our codebase where we need to handle conditional filtering in ActiveQuery
relationships. The original implementation used a traditional if-statement approach, which while functional, wasn’t elegant as it could be. Here’s where Yii2’s filterWhere
method came to the rescue.
The original code looked something like this:
public function getPlantParameters(): ActiveQuery
{
$query = $this->hasMany(PlantParameter::class, ['idEmission' => 'id']);
if ($this->operationalConditionId === null) {
return $query;
}
$tableName = PlantParameter::tableName();
return $query
->andWhere(["$tableName.[[idOperationalCondition]]" => $this->operationalConditionId])
->addOrderBy(["$tableName.[[order]]" => SORT_ASC]);
}
By leveraging filterWhere
, we simplified this to:
public function getPlantParameters(): ActiveQuery
{
$tableName = PlantParameter::tableName();
return $this->hasMany(PlantParameter::class, ['idEmission' => 'id'])
->andFilterWhere(["$tableName.[[idOperationalCondition]]" => $this->operationalConditionId])
->addOrderBy(["$tableName.[[order]]" => SORT_ASC]);
}
This optimization eliminated the need for explicit null checking, reduced the code by several lines, and made the logic more straightforward. The filterWhere
method could automatically handle empty values by ignoring them in the query condition, leading to cleaner, more maintainable code.
These kinds of optimizations might seem small, but they reflect our commitment to continuous improvement. We’re always looking for ways to make our code more elegant and efficient, even if it means revisiting and refining existing solutions.
If you’re working with Yii2, take a look at your query builders – you might find similar opportunities to simplify your code using filterWhere. It’s a powerful feature that’s often overlooked but can make your codebase significantly cleaner.
Have a complex Yii2 project that needs optimisation? Let’s connect! Reach out to us to explore how we can help improve your codebase efficiency.
Photo by Alternate Skate on Unsplash